Active Computers & Programming Posts

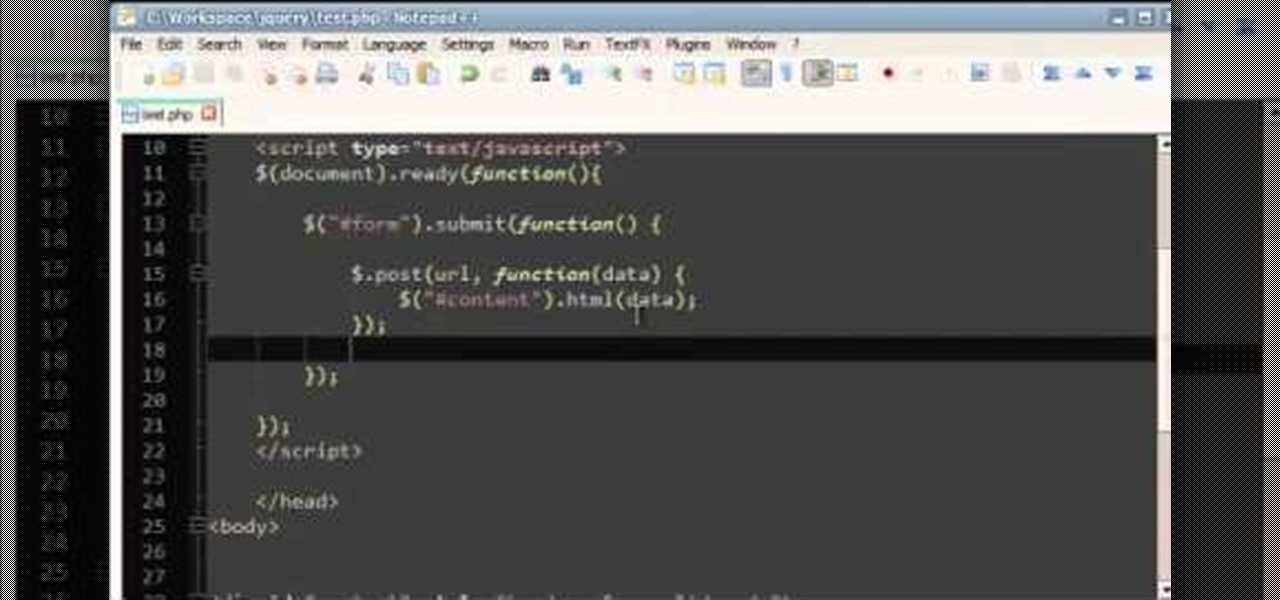
How To: Submit data to your server using JQuery and AJAX
This tutorial is a quick introduction to the $.post() function when you're using JQuery. This function is most often used to submit data to your website server, and is also a useful function when you're programming in AJAX as well.
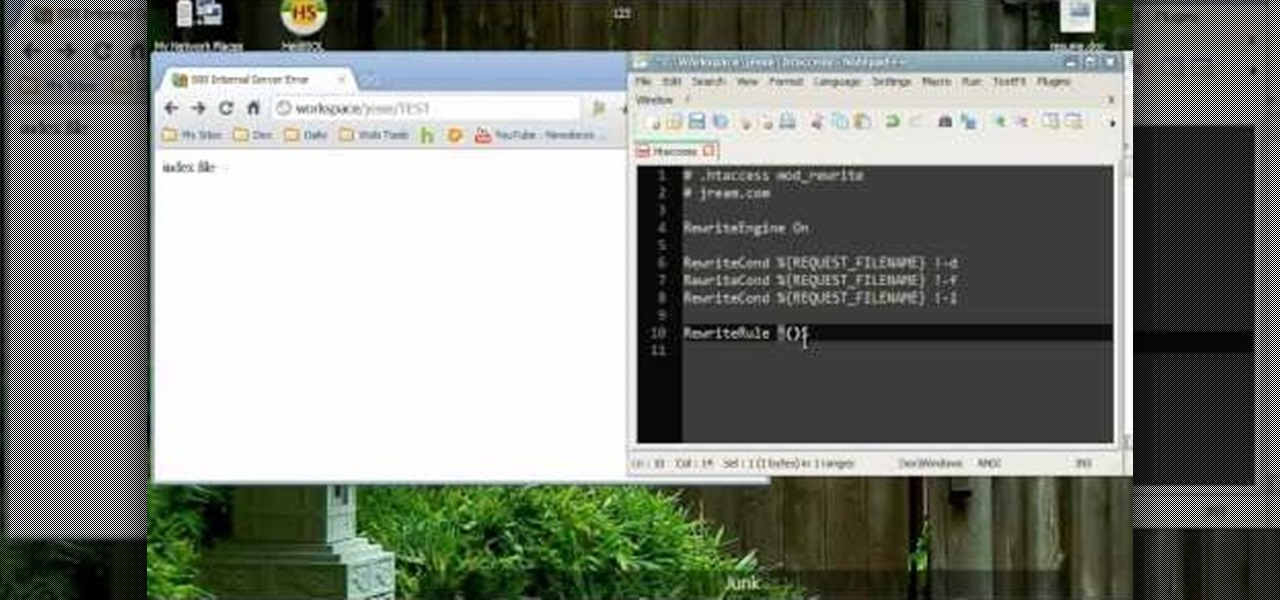
How To: Get around in Mod Rewrite and master some of the basics
First, turn Mod Rewrite on (which it usually is). Once it's on, then you can follow this tutorial to learn how to master some of the basics of Mod Rewrite. Keep easy track of all your files on your Windows based machine.
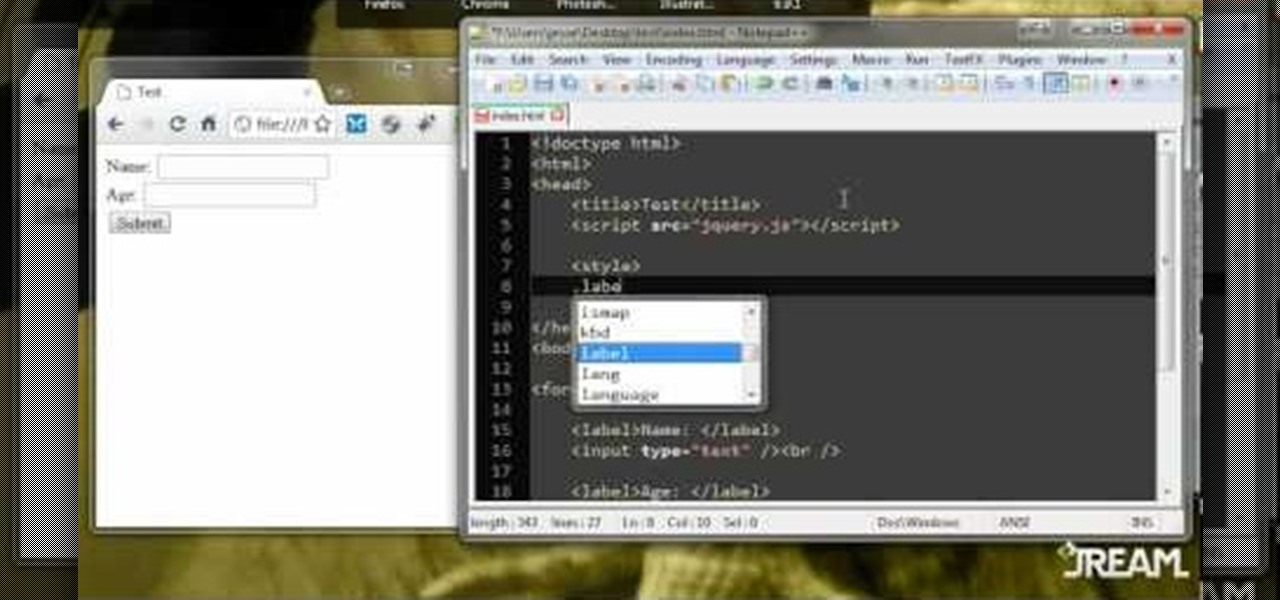
How To: Fake an AJAX-looking form using JQuery
This form will look like it's been written with AJAX, but the dirty little secret is that it's actually a JQuery script. The reason for doing it this way is greater efficiency in your program, and a little easier to work with.
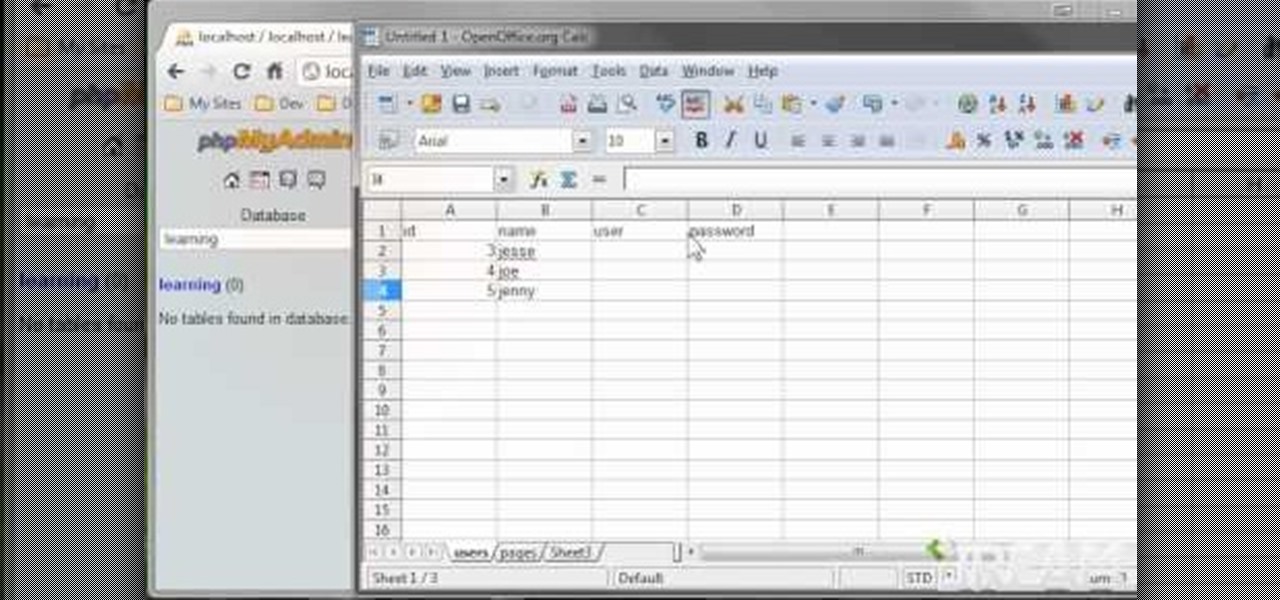
How To: Get everything you need to start teaching yourself MySQL
MySQL is a structured query language (hence the 'sql'), and is an open source language published by Oracle. If you want to learn how to manipulate and use databases (such as customer lists or membership rosters), you will need to learn MySQL.
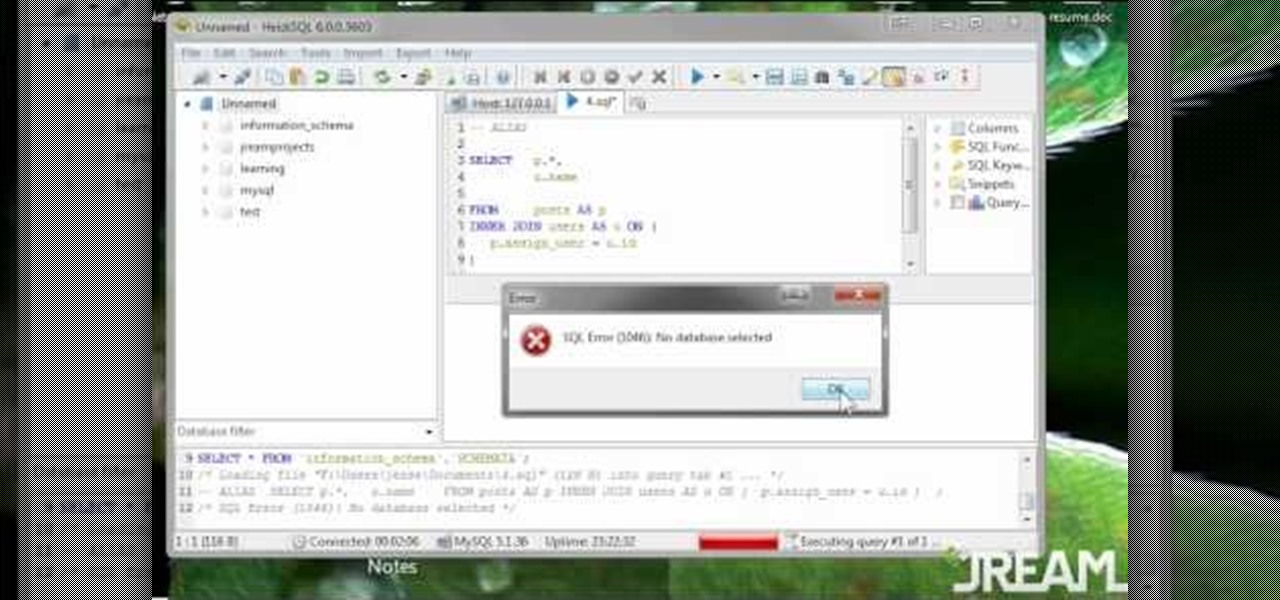
How To: Use the ALIAS command in MySQL as shortcuts in your programming
The greatest function of the ALIAS command is as a shortcut. Being able to use this properly will make your MySQL database much easier to use, more functional and streamline all your programming. This tutorial shows you everything you'll need to know about using ALIAS in your database programs.
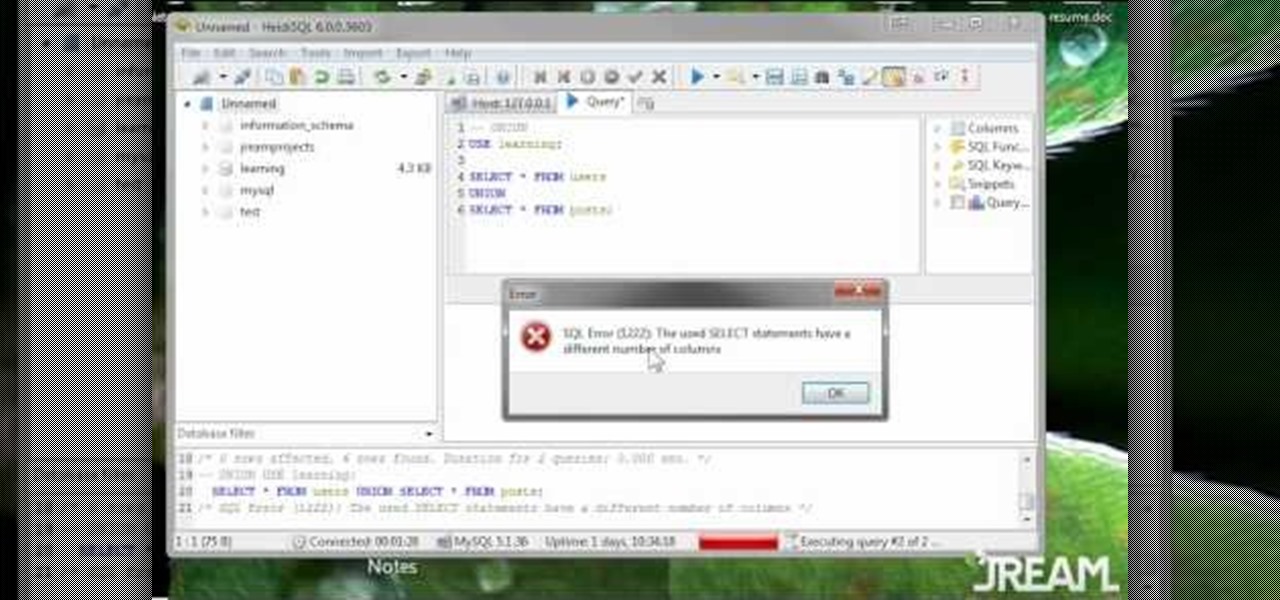
How To: Use the UNION command when working in MySQL
When you use the UNION command, you are stacking one result set on top of another. This is slightly different from the JOIN command, and this video is here to explain what the difference is and the best way to use UNION in your own MySQL database programs.
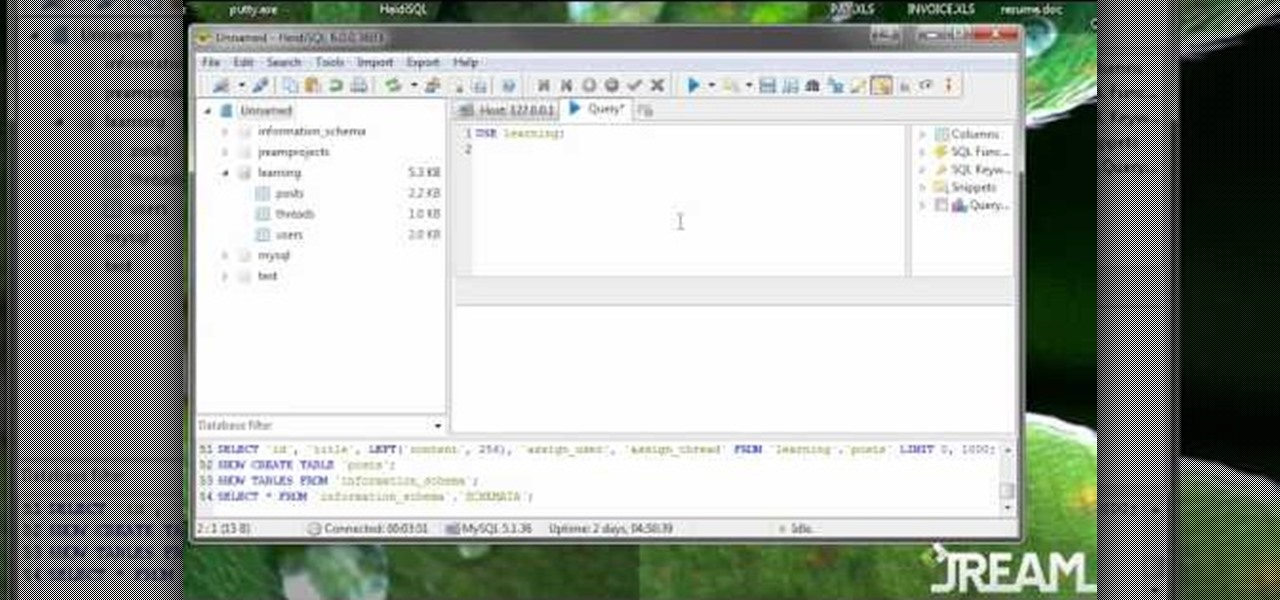
How To: Write a subquery or sub select in your MySQL database
Wait, a query within a query? This function is possible within the framework of MySQL. Learn how to correctly implement a subquery or sub select in your next database project and avoid a couple perils that will make your program unstable.
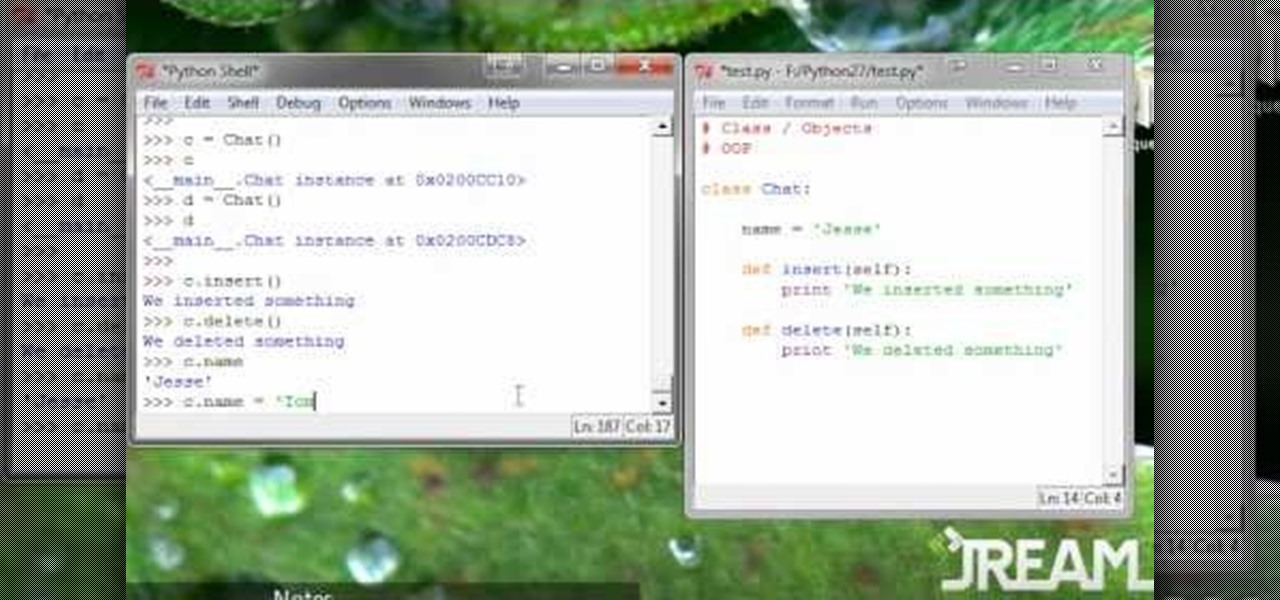
How To: Use classes and objects when coding a program in Python
You'll need to master the basics of object oriented programming to be able to use Python, and that's what this tutorial is all about. Use classes and objects so you can make your programs as efficient and elegant as possible when writing in Python.
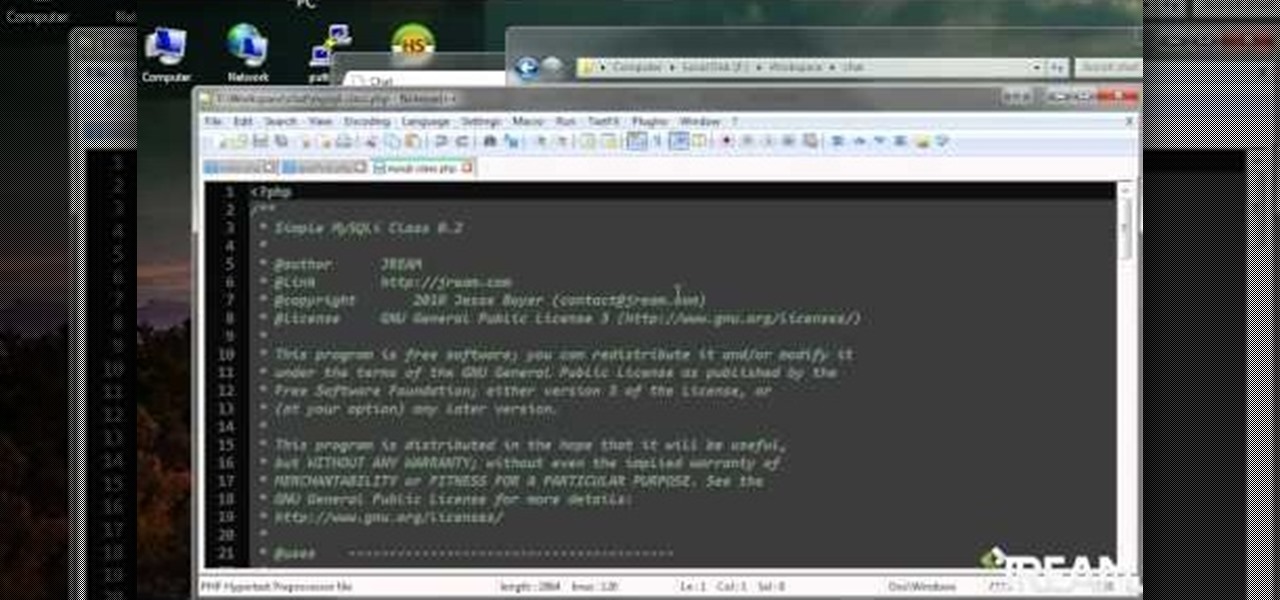
How To: Make a chat box on your website using JQuery, MySQL and PHP code
This tutorial shows you how to set up an interactive chat box on your website, so you can allow members to communicate with each other in real time. It can be a bit tricky to master, but will definitely be worth it for your site in the long run.
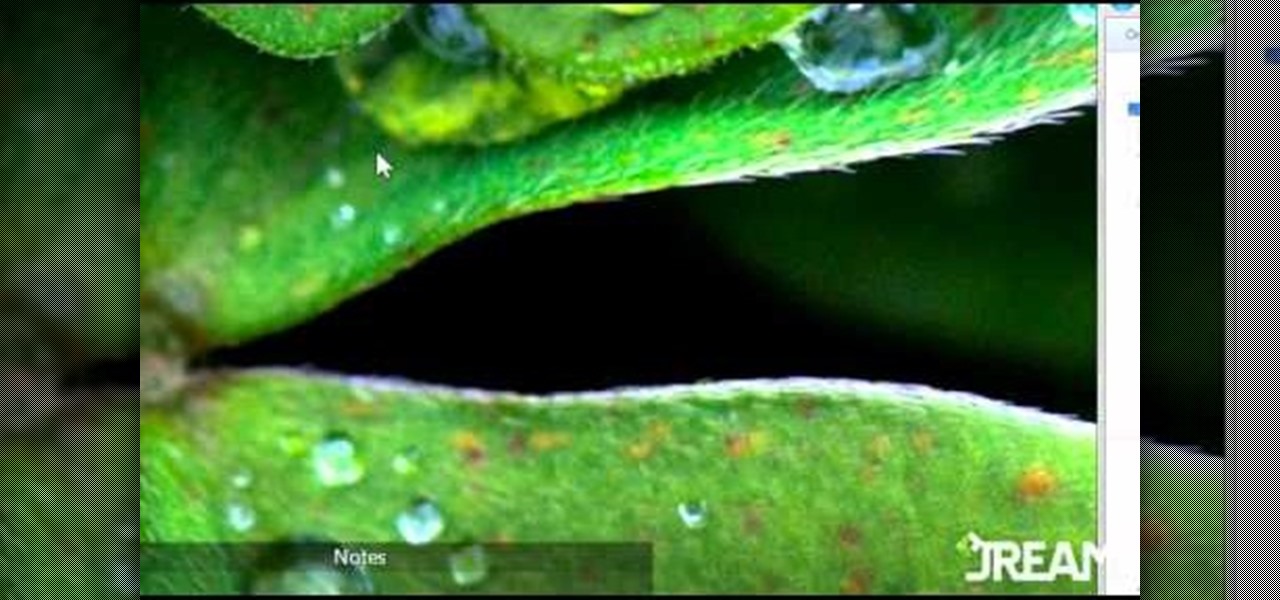
How To: Set up a command shell in your Python based program
Of course, you'll want other people to be able to interact with your program. Linux sets up a command shell automatically, but if you're programming with Python on a Windws machine, you'll need to do it manually - and this video shows you how.
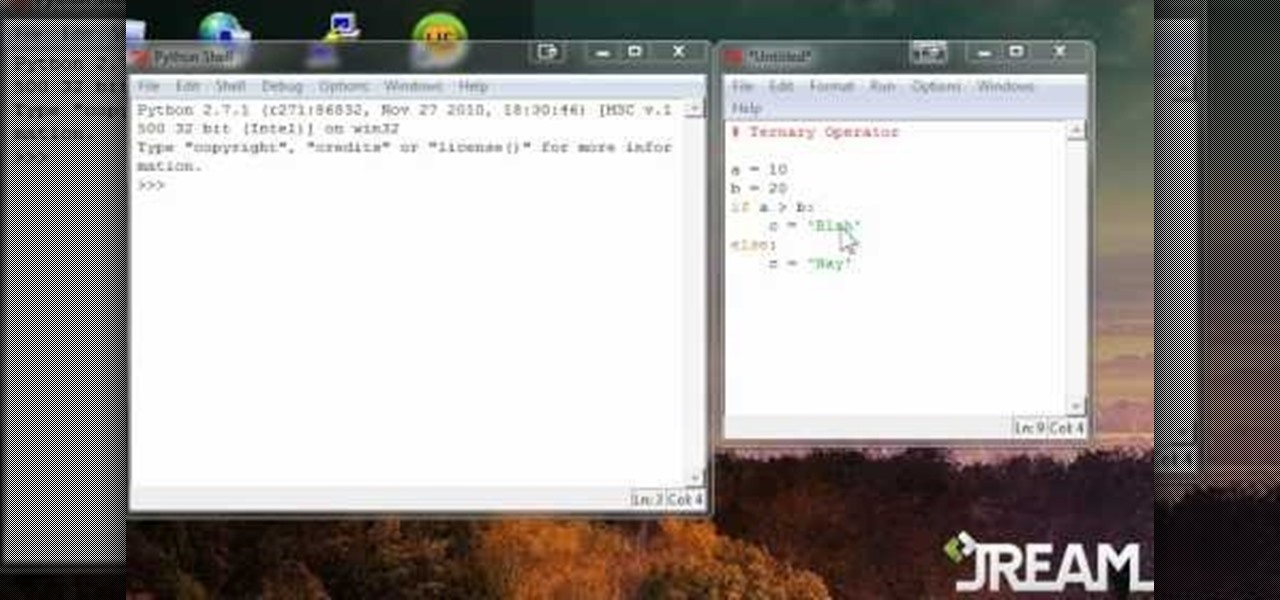
How To: Use the ternary operator to streamline your programming in Python
A ternary operator lets you smooth out your Python based program. This video shows you precisely how one works, why it's a good thing and how you can incorporate a ternary operator to your own Python based computer programming projects.
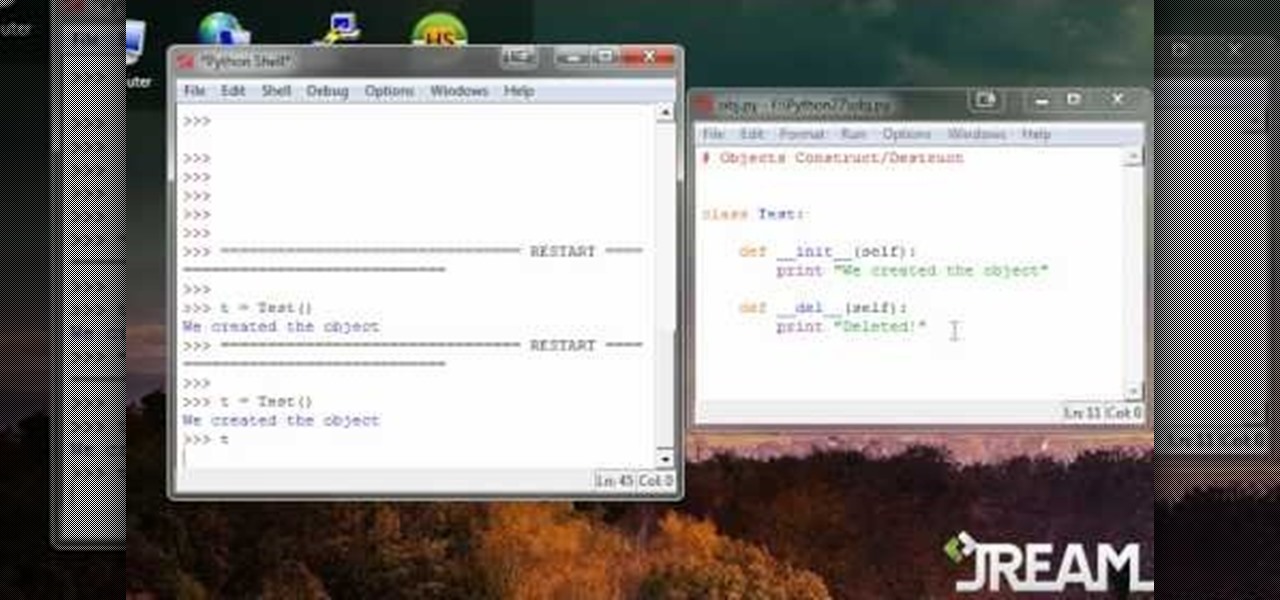
How To: Construct or destruct an object when you're writing code in Python
If you already grasp the basics of object oriented programming, this tutorial will be very easy for you. When you construct an object, you create it within the program (in this case, a program using Python), and when you destruct it, it deletes.
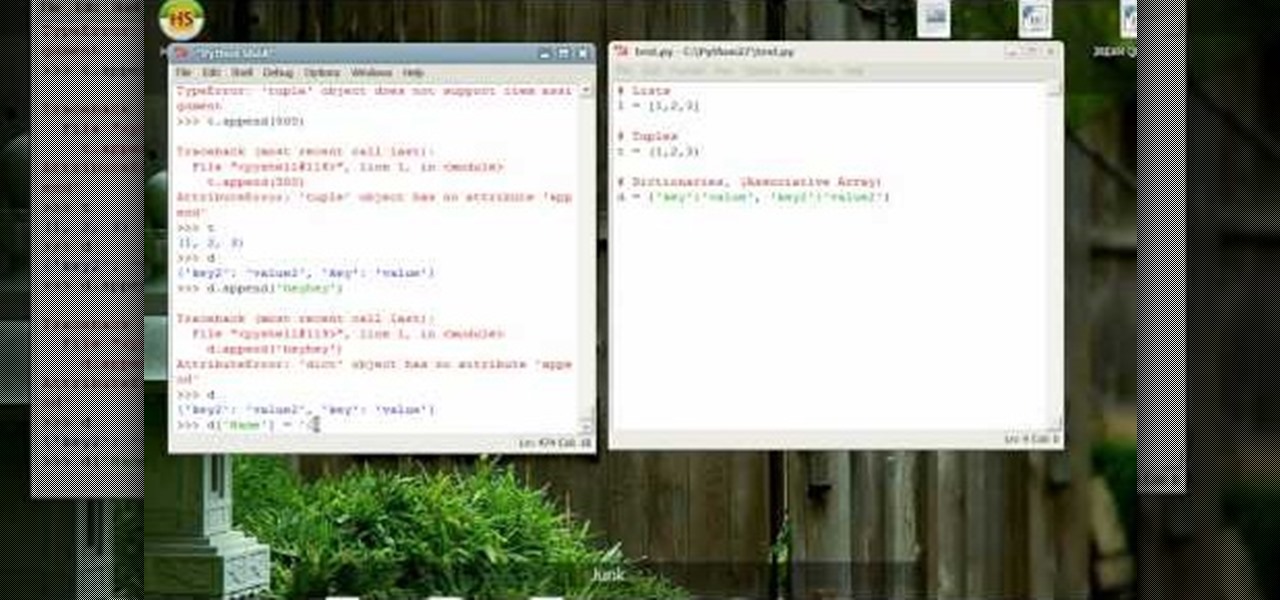
How To: Use tuples and dictionaries when programming in Python
A tuple's value cannot be changed at all within your Python-based program, whereas a dictionary is more like an associative array where every item has a key and a value. Here is how you can use both tuples and dictionaries within your Python program.
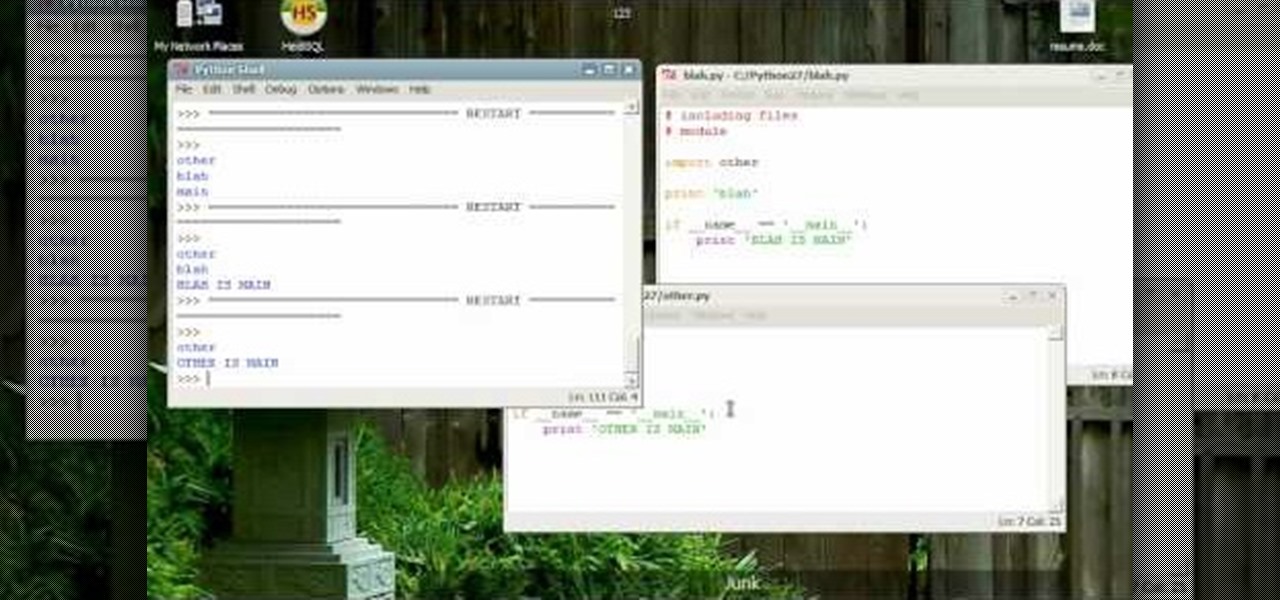
How To: Include a file or module with your Python program
Including a file (also called a module) is easier in Python than in some other common programming languages. This video shows you how you can take a small, basic file and then incorporate it into your Python based computer program.

How To: Write your first Hello World program using Java
Whether you're an experienced programmer looking to add another language, or completely new to computer language and wanting to start with Java, this program is for you. After you've downloaded and installed your SDK, follow this tutorial to write your first Hello World program.

How To: Use variables when writing a program in Java
Java works a little differently than languages like Python or PHP when it comes to working with variables. This tutorial shows you what the difference is, how you can define your variables and the easiest ways to work with them when writing programs in Java.

How To: Use objects and object methods when programming in Java
In Java, everything is an object, which can make it a little scary to start programming using this language. But that's what this tutorial is for! Here is how objects and object methods work when you're designing a program with Java.
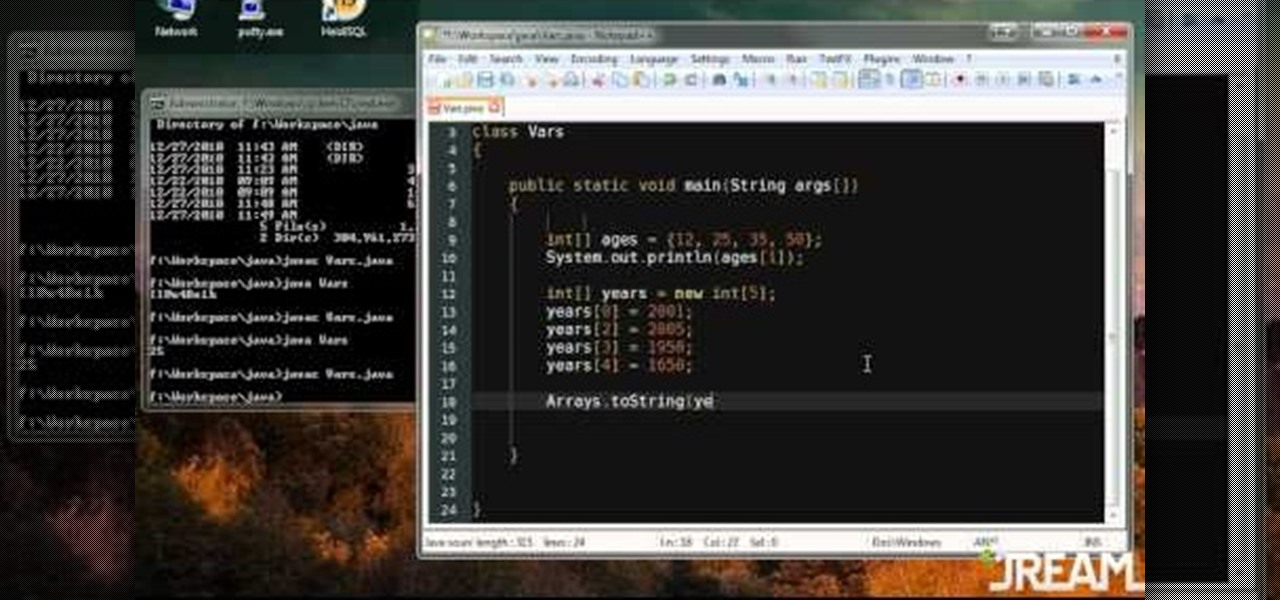
How To: Incorporate arrays into your programs written in Java
Arrays are little pockets of data distributed throughout your program. They're useful because having arrays keeps you from needing to work with hundreds of variables when programming. This specific tutorial shows you how to work with arrays when you're using Java to program.

How To: Create a custom HTML image map for your blog or website
First, make sure that your image is hosted on a secure place online (such as your own Photobucket account). Then go to ImageMaps.com and use this free site to create your own image map that can now be added to your website.
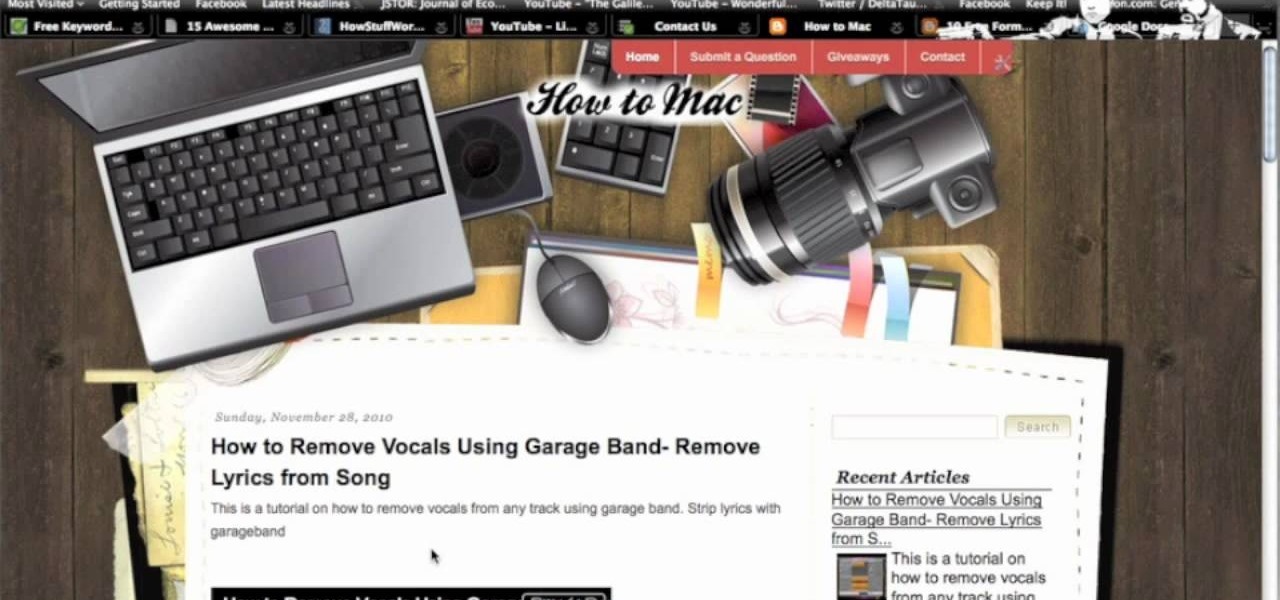
How To: Create a web form using Google Docs and embed it on your website or blog
Start with the free Google Docs, which you can get with any Gmail account. Then get one of the free web form templates (this video uses the Contact Us form as an example) and then edit and embed it on your own website.
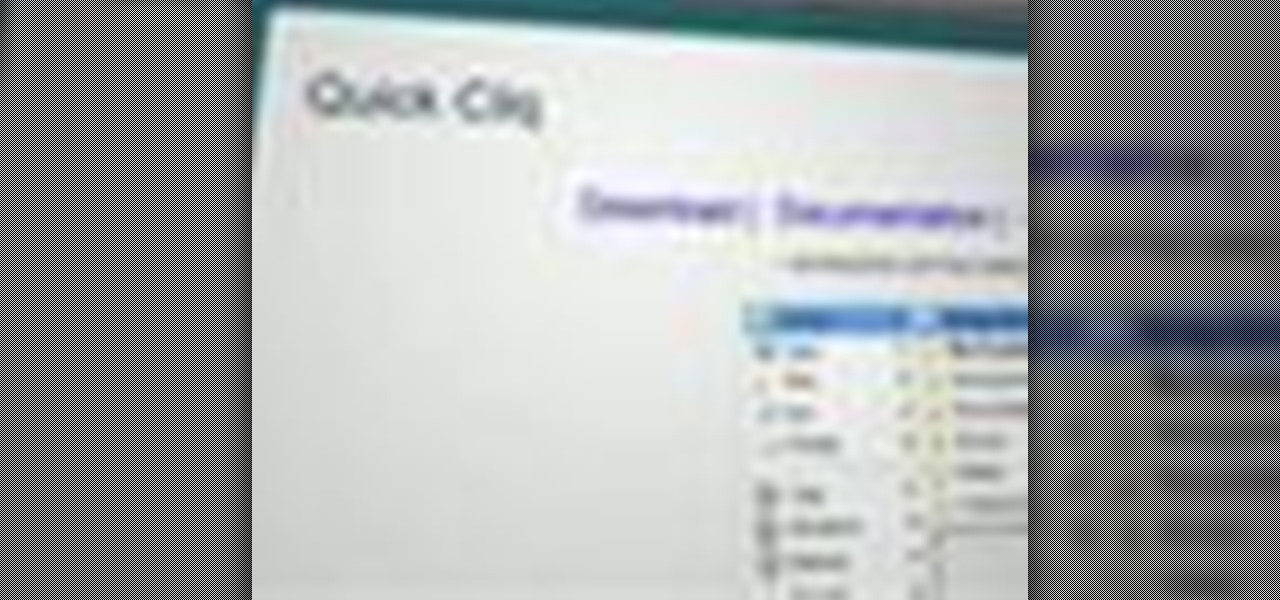
How To: Get one-click access to Windows 7 files and folders using Quick Cliq
If you're trying to streamline your work on your Windows 7 PC, here's a handy little program to help you speed things up. Quick Cliq lets you you customize your Windows startup menu to give you one-click access to files, folders applications.

How To: Launch apps from the Mac OS X menu bar using HimmelBar
View all your installed applications on your Mac quickly and easily using the HimmelBar. This free program adds a new icon to your menu bar which provides instant access to all those programs with a simple click of a button.
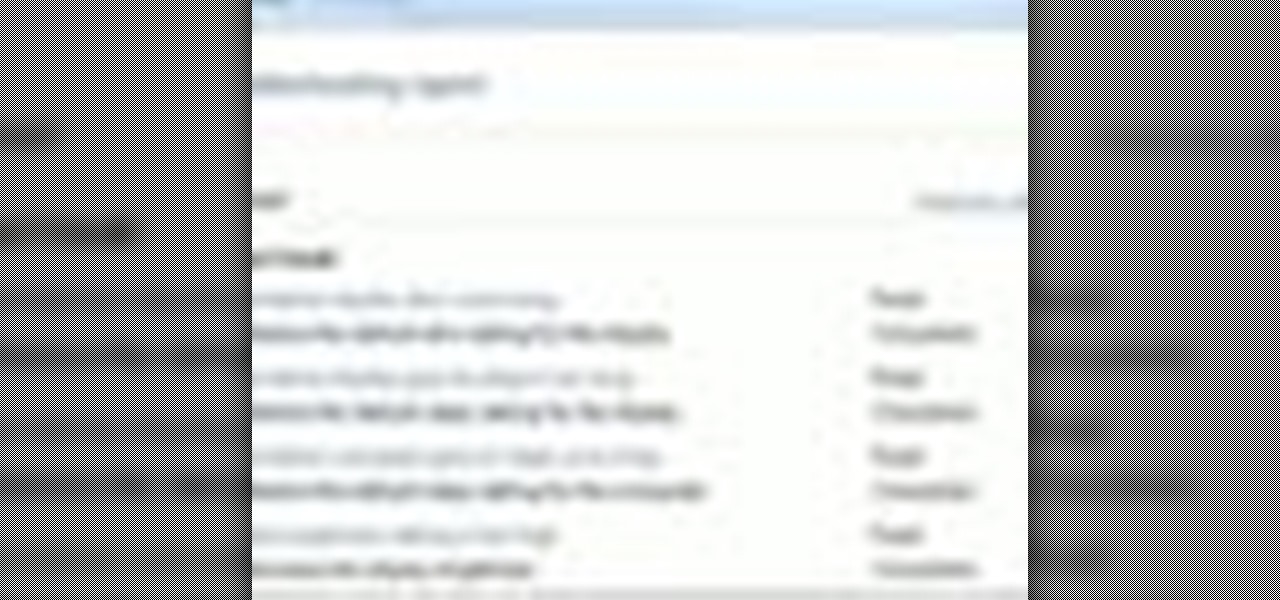
How To: Get the most out of your Windows 7 laptop battery
Going, going... gone! Don't let your Windows 7 laptop battery go down on you. With this quick and helpful video, learn how to control your laptop's battery settings via Windows 7's power usage function to ensure you get every last drop of your battery's power.

How To: Use the ATI Overdrive utility to overclock your ATI AMD Radeon Graphics Card
Are you a speed freak? Not getting the fps rate you want in your favorite 1st person shooter? If you're answering yes, then it's time to get overclocking. This step-by-step video shows you how to use the ATI Overdrive utility and the MSI Afterburner utility to overclock your ATI AMD Radeon Graphics card to push those pixels to the max! The MSI Afterburner utility works with both Nvidia and ATI cards.
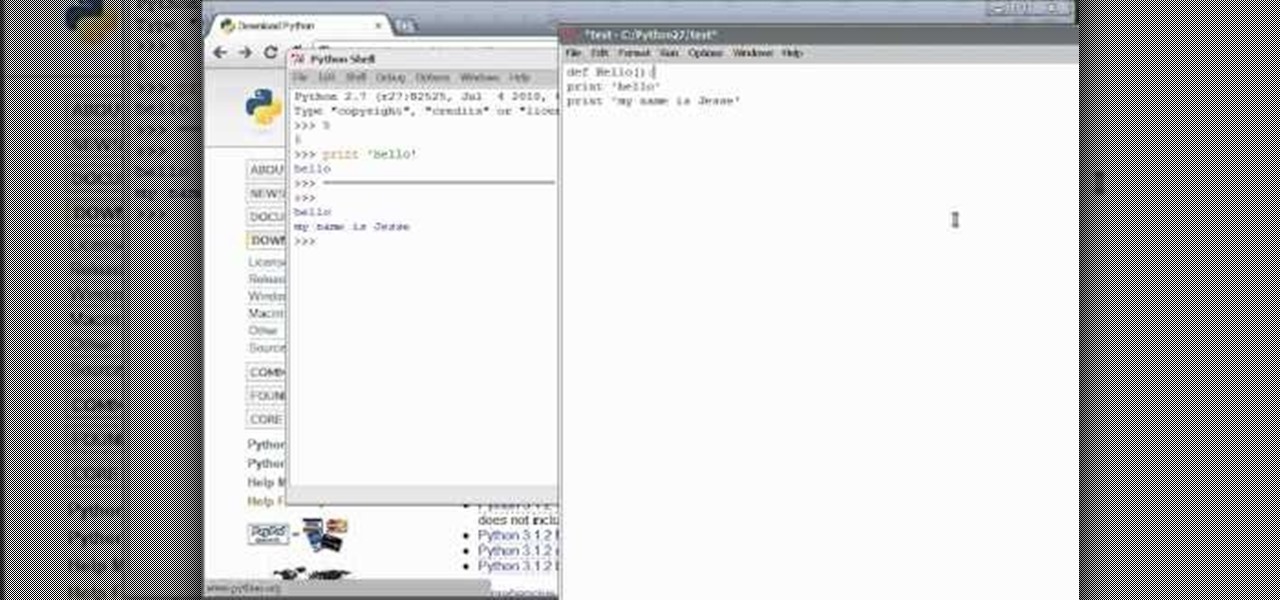
How To: Install a compiler and start programming in Python
Python can be used for games, websites and operating system GUIs; and you can work with it on PCs, Macs or Linux machines. This tutorial shows you how to install the compiler and get started teaching yourself how to program in Python.
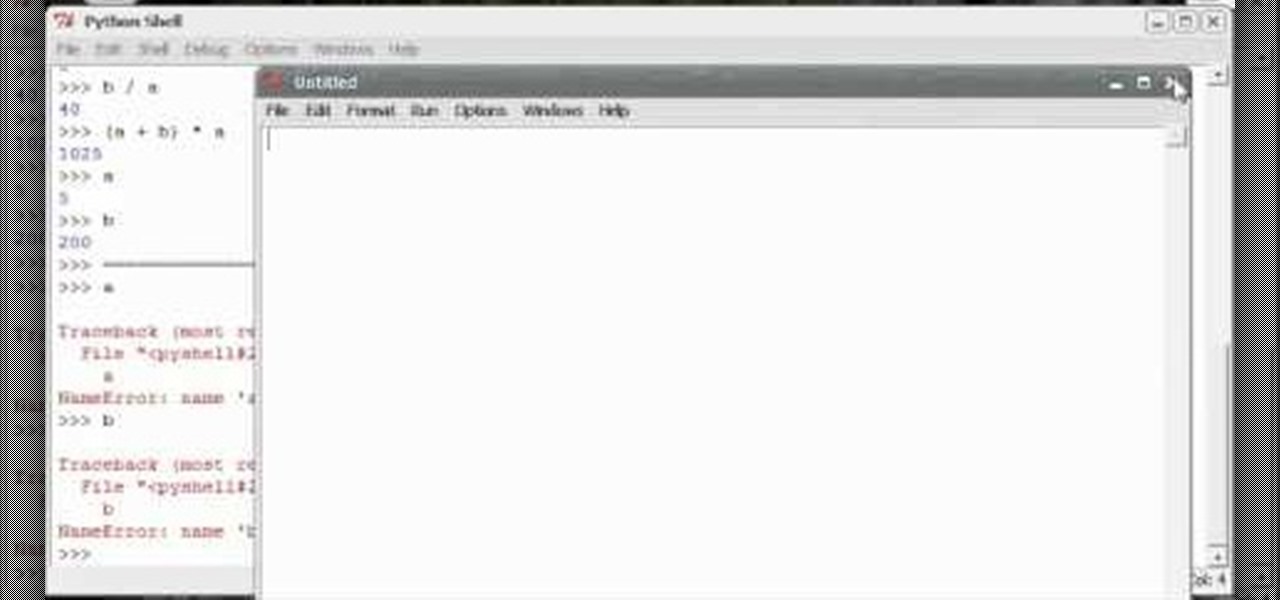
How To: Use variables when programming with the Python language
Variables are the core of nearly any programming language, and Python is no different. This tutorial shows you how to work with variables when you're starting to program in Python. This also shows you how to get familiar with the shell in Python.
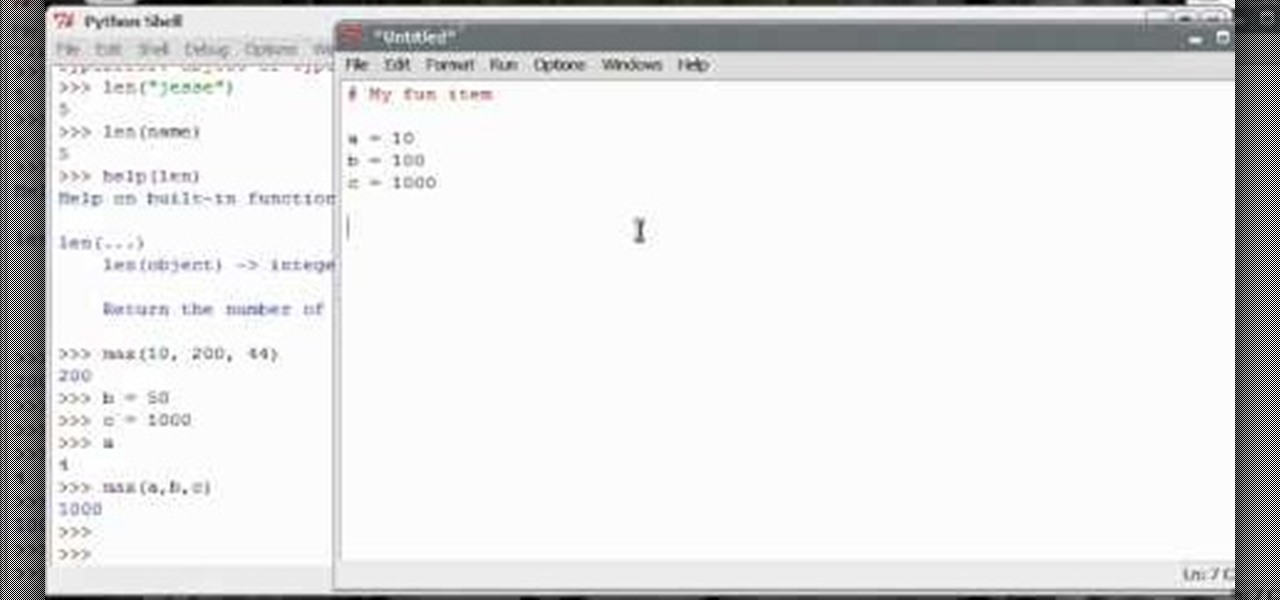
How To: Use the basic functions in Python and import modules
So you've mastered variables, and now it's time to take a look at functions and how they work on the very basic level in Python. This tutorial also takes a brief look at importing modules, and how they work in your Python program.
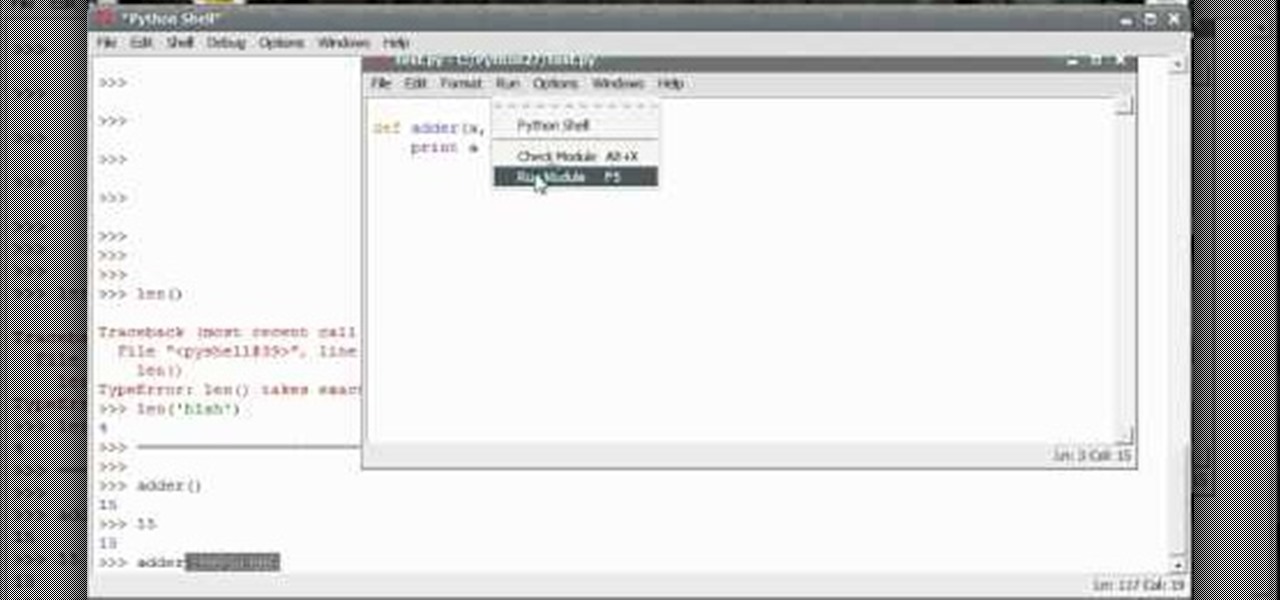
How To: Create and use your own functions when programming in Python
Functions allow you to repeat particular actions without having re-code them for every instance. This tutorial shows you how to set up and use your own functions when you're writing a program using Python. Make sure all your functions are well defined before you start using them!
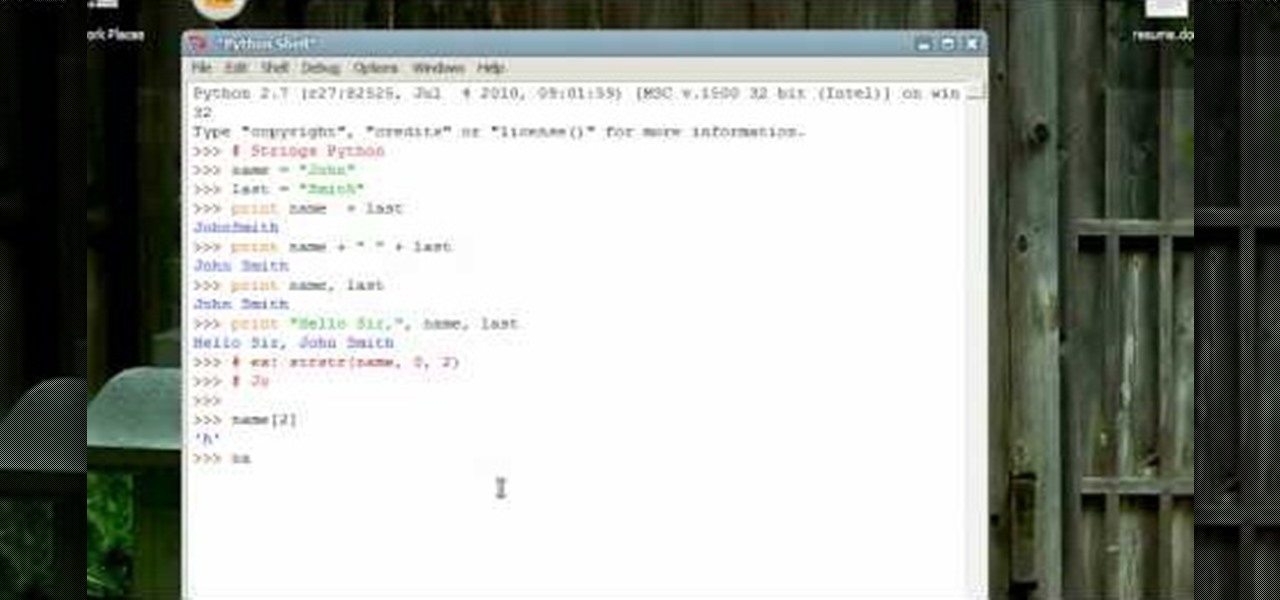
How To: Use strings and slices when programming in Python
So you've already mastered variables and functions - now it's time to progress to strings, substrings and slices when you're working on a program using the Python programming language. Python strings are much less complex than in other languages, which makes them easy to use.

How To: Use string methods and object methods when programming in Python
When you're programming in Python, and make a variable into a string, that becomes an object. This tutorial shows you how to use string methods and object methods when you're teaching yourself how to write a program using the Python language.

How To: Use loops when writing a program in Python
This tutorial shows you the two basic types of loops in Python - While and For. Be careful that you don't accidentally write an infinite loop, but still be able to use them to increase the efficiency of your programs.
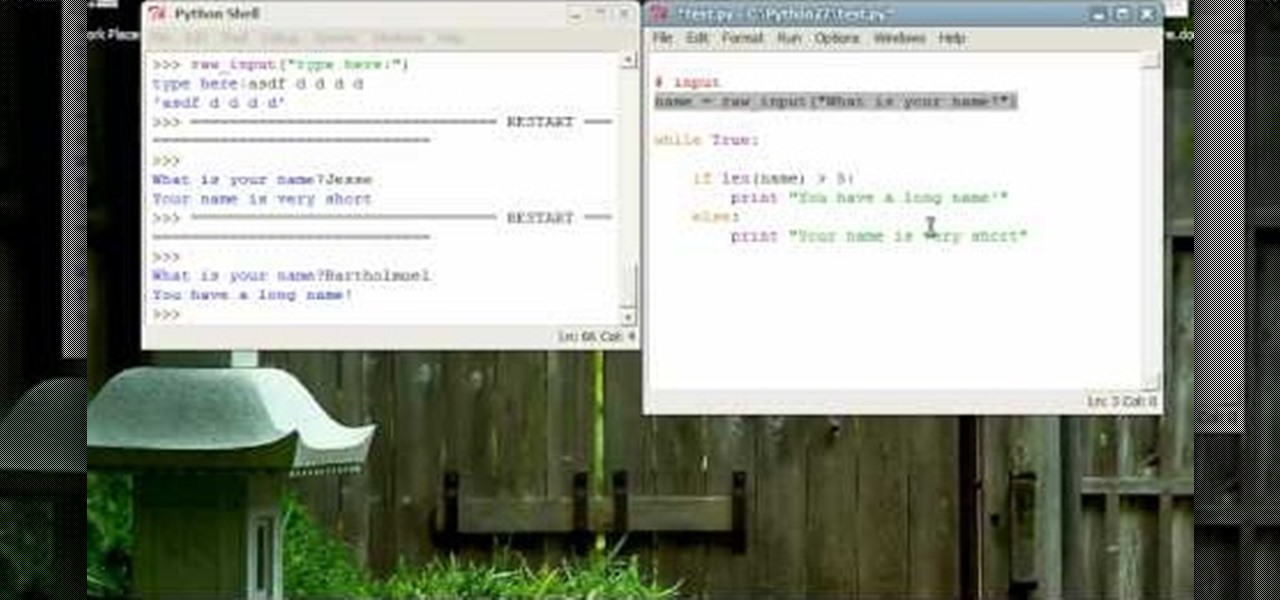
How To: Incorporate user input into your Python program
Now that you've mastered the nuts and bolts of Python, it's time to add in another layer of complexity - allowing for user input to your program. Write a small dice rolling program using all the principles you've studied so far.
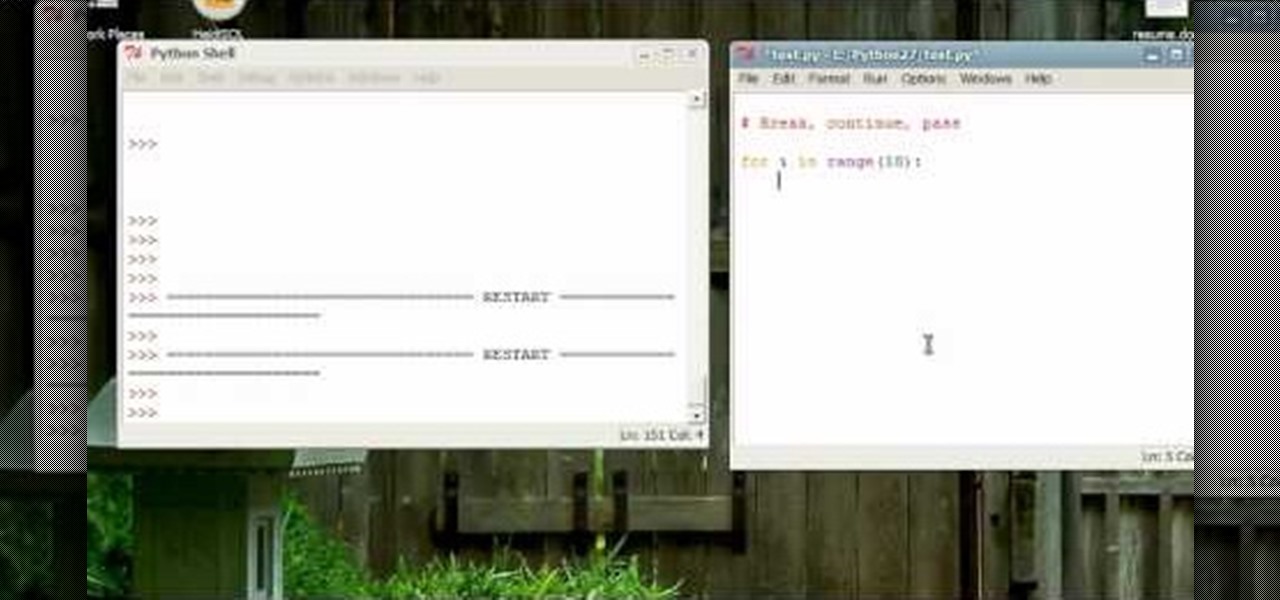
How To: Use the break, continue and pass tricks in Python loops
If you've learned other programming languages, you're likely already familiar with the break and pass flow control commands when programming loops. This video shows you how to use them when writing code in Python, and also how to use the continue trick in your program.
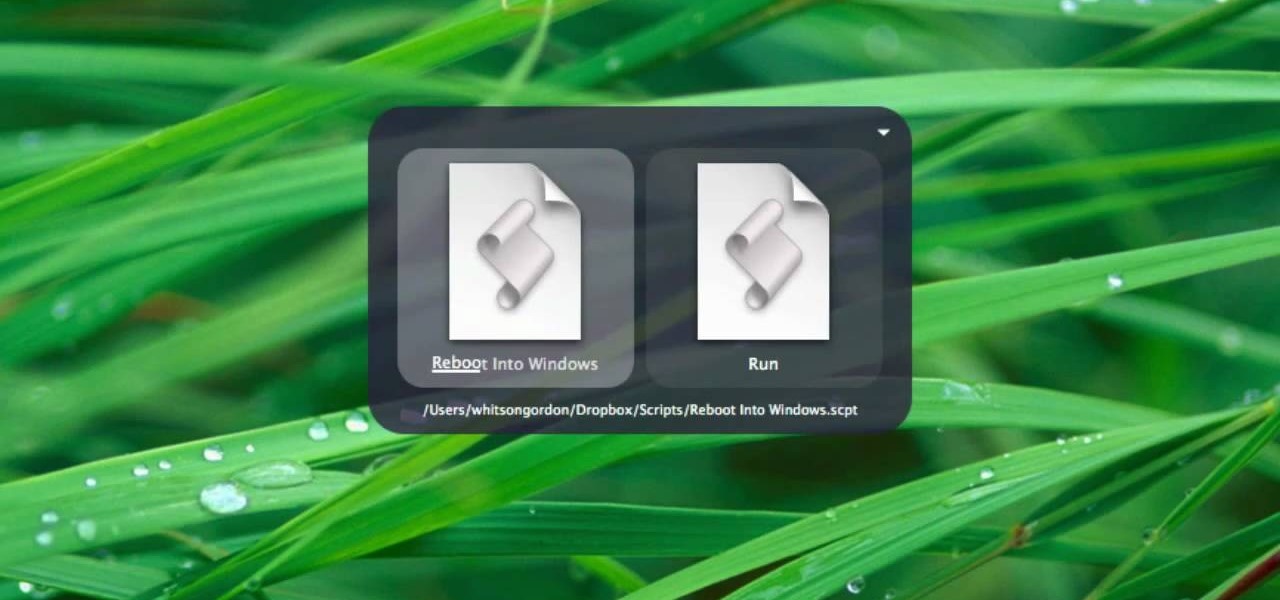
How To: Use simple AppleScript & Quicksilver to boot your Mac into Windows
So are you a Windows or a Mac? Well, some days you may be one, and other days you may be the other, SO, this handle little how-to will show you how to use Quicksilver to boot up into either OS whenever you want.
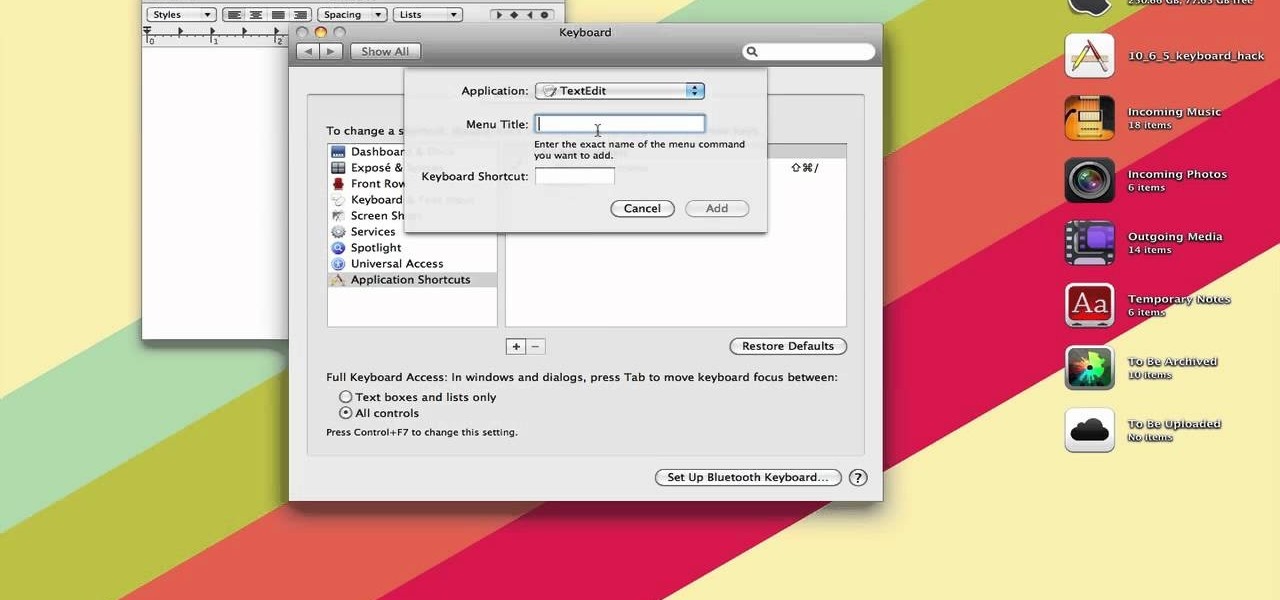
How To: Customize your keyboard shortcuts in Mac OS X
If you're a Mac user, you know the keys to the castle are literally knowing how to use the shortcuts and hot-keys. But, what if you don't like the predefined OS X setup? Well, simple answer, change them. This is a quick guide to remapping any keyboard shortcut in any application running in Mac OS X.
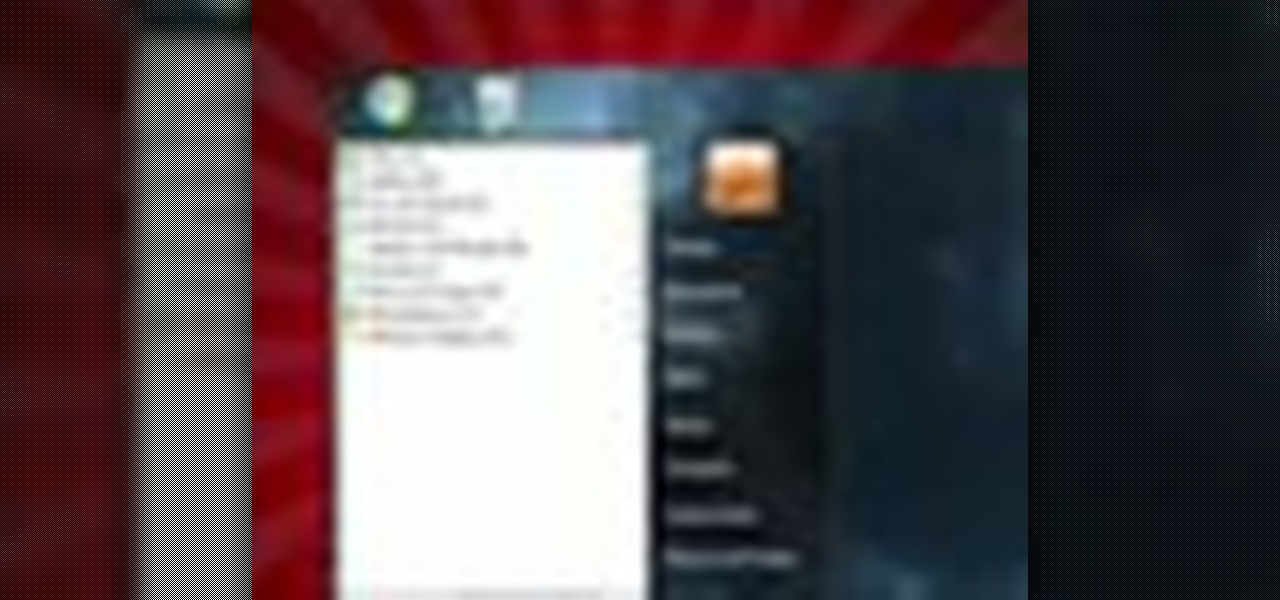
How To: Customize the layout of your Windows 7 start menu with Handy Start Menu
If you need to take control of your Windows 7 start menu, you're going to want to watch this. Step-by-step instructions on how to personalize your start menu with a nifty little program called, Handy Start Menu. Then, you can see the programs you want, and hide the programs you don't want to see. That IS handy!
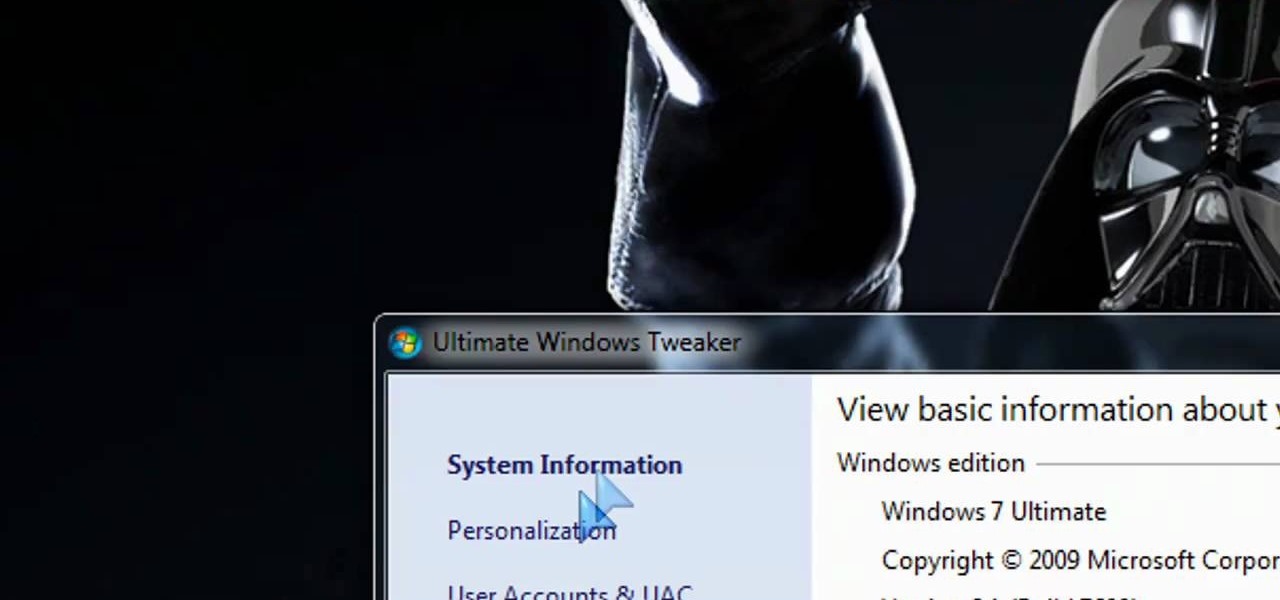
How To: Install and run Ultimate Windows Tweaker for Windows 7 & Vista
If you're not satisfied with your out-of-the-box version of Windows 7 or Vista then get ready to tweak it up good! With this video, you'll see how to use a little program called Ultimate Windows Tweaker to wrestle your software to perform the way YOU want it to. This software lets you get into the registry in a safe and easy way if you're not a computer wizard. Remember to always back up your system before toying with the registry.
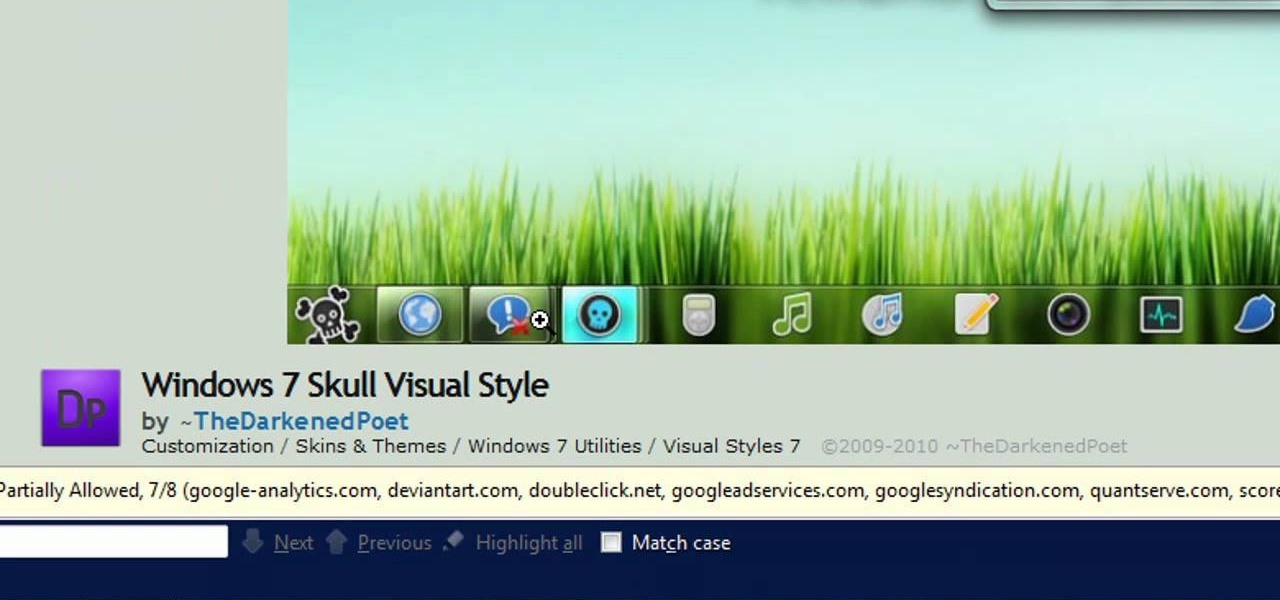
How To: Use UxStyle to customize your Windows 7 themes and styles
If you want to take control of the themes and styles of Windows 7, you're going to want to get your hands on a little program called UxStyle. This program works for 32 and 64 bit systems and gives you great control over the look of your system. Don't forget to back eveything up first!
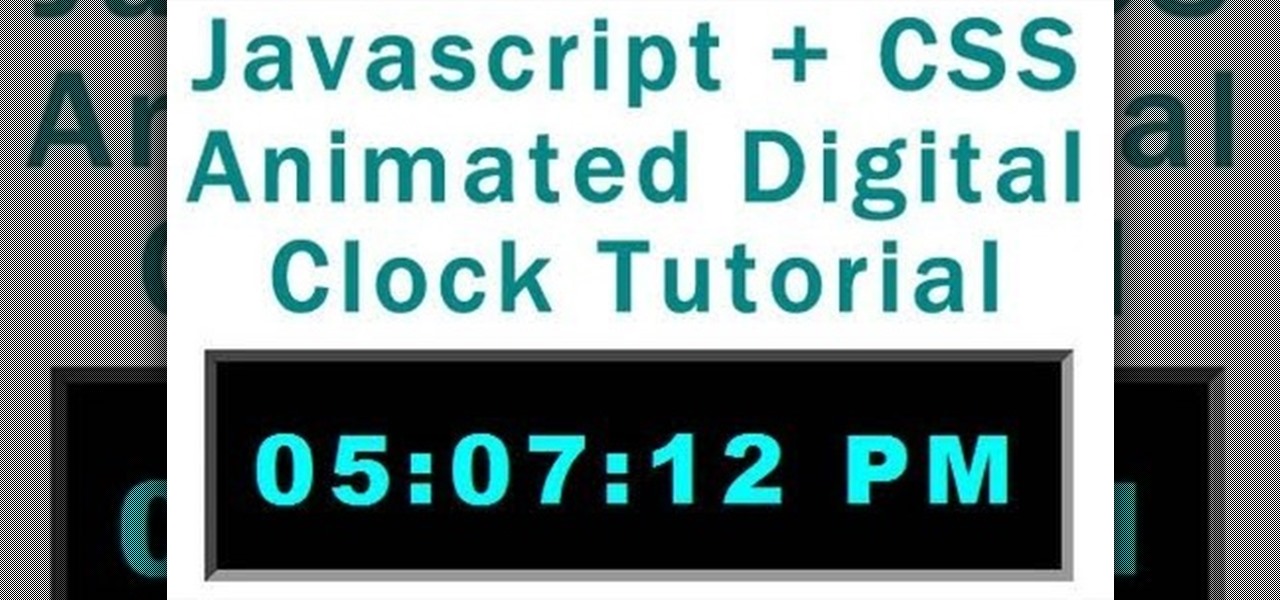
How To: Make an animated digital clock for your website using Javascript CSS
Need to make a custom, digital clock that will run in all major desktop and smartphone browsers? This tutorial shows you how to employ Javascript functions to communicate in real time with web page elements to get a slick CSS animated clock. Cool, eh?
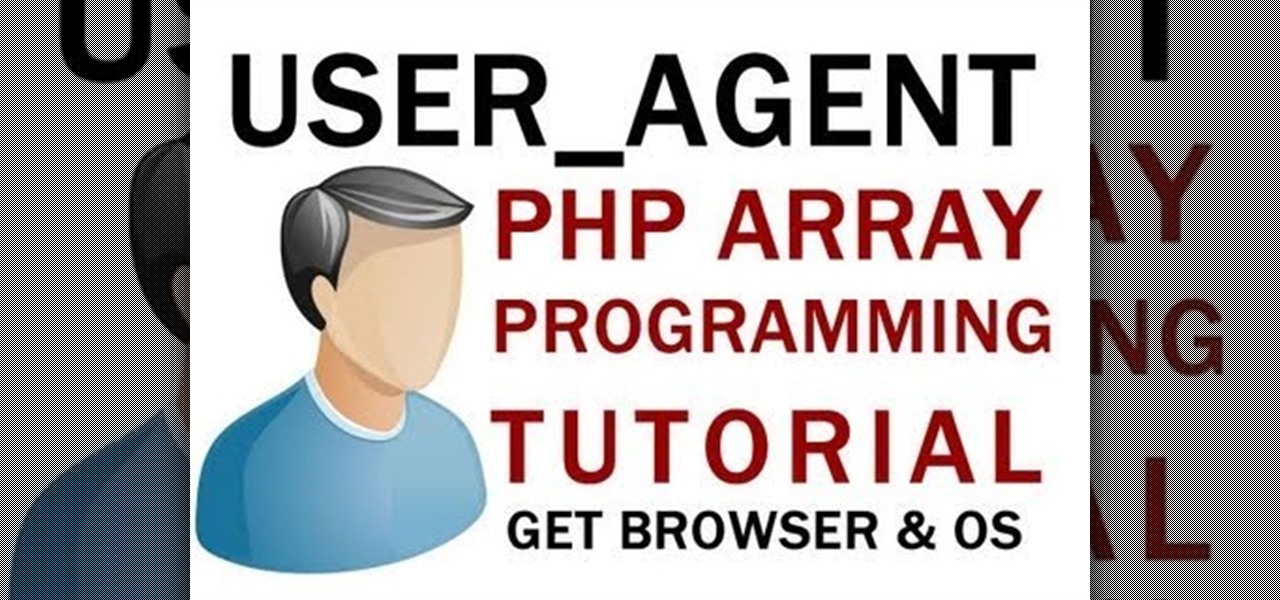
How To: Use PHP array programming to make a user agent sniff script
OK, so visitors are coming to your website and they're even leaving comments! Now, with some simple PHP array programming you can find out what browser and OS they're using. This tutorial shows you how to create a simple and efficient user agent sniff script to get the information you want. Sniff, sniff. Is that Chrome running on OS X I smell?